Overview
Morpheus is a desktop application that aims to facilitate the administrative work of hotel services as a hotel management system. The user interacts with it using a CLI, and it has a GUI created with JavaFX.
Summary of contributions
-
Major enhancement: Set up the billing system for the Morpheus
-
Added the ability to charge services to a bill, remove charged services from a bill and fetch & view bills
-
What it does: Allows the user to keep track of guests' bills and outstanding payments, with a list of their requested or used services.
-
Justification: This feature improves the product significantly because as a hotel management system, Morpheus should be able to carry out administrative work like bookkeeping. This makes the billing system a key feature of the product.
-
Highlights: This enhancement is a core feature of Morpheus and necessary feature of the billing system. Hence, it affects commands to be added in the future. It required an in-depth analysis of design alternatives as there were many ways to keep track of and manipulate bills in the code.
-
-
Added the ability to store & load bills
-
What it does: Allows the application to store bill information to the hard disk upon exit and load the data when reopened.
-
Justification: This feature improves the product significantly because in order to facilitate bookkeeping, Morpheus must be able to save and load bill information.
-
Highlights: This enhancement is a necessary feature of the billing system and therefore affects commands to be added in the future. It required a deep understanding of the storage component in the original addressbook.
-
-
-
Minor enhancement: added the ability to set the cost of a room.
-
Code contributed: [Functional & Test code]
-
Other contributions:
-
Project management:
-
Managed releases
v1.2
-v1.4
(3 releases) on GitHub -
Maintaining the issue tracker
-
-
Enhancements to existing features:
-
Documentation:
-
User Guide: #95, #177, #183, #270
-
Standardized and cleaned up team’s updates
-
Added
setrcost
,chargeservice
,deletecservice
&fetchbill
features -
Updated Introduction, Command Summary
-
-
Developer Guide: #95, #259, #271, #278
-
Standardized and cleaned up team’s updates
-
Added Billing System Implementation
-
Added diagrams (Billing System)
-
Updated Introduction, User Stories, Use Cases
-
-
-
Community:
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Setting the cost for a room : setrcost
(Hui Ming)
If you want to set the cost for a room (per night), use the command setrcost
Format: setrcost rn/ROOM_NUMBER c/COST
Examples:
-
setrcost rn/101 c/50.00
Result: Sets the cost for 101
as 50.00
per night.
Charging a service : chargeservice
(Hui Ming)
If you want to charges a service to the guest’s tab, use the command chargeservice
Format: chargeservice i/PERSON_ID rn/ROOM_NUMBER si/SERVICE_ID
Examples:
-
chargeservice i/G1231231X rn/100 si/WC
Result: Charges service with the ID WC
for room 100
to the guest with ID G1231231X
's bill.
Deleting a service from a bill : deletecservice
(Hui Ming)
If you want to remove a charged service from the guest’s bill.
Format: deletecservice i/PERSON_ID rn/ROOM_NUMBER si/SERVICE_ID
Examples:
-
deletecservice i/G1231231X rn/100 si/WC
Result: Removes service with ID WC
from guest with ID G1231231X
's bill for room 100
.
Fetching bill of a guest : fetchbill
(Hui Ming)
If you want to retrieve the bill of a guest, use the command fetchbill
Format: fetchbill i/ID [rn/ROOM_NUMBER]
Examples:
-
fetchbill i/G1231231X
Result: Shows the entire bill, consisting of all costs incurred, for guest with ID G1231231X
's stay up till present moment.
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Introduction
Product Description (Hui Ming)
Morpheus is a desktop application that aims to facilitate the administrative work of hotel services as a hotel room management system. The system will also provide statistics and reports, constructed from the analysis of the details in the user-provided database. Most of the user interaction occurs via a CLI (Command Line Interface).
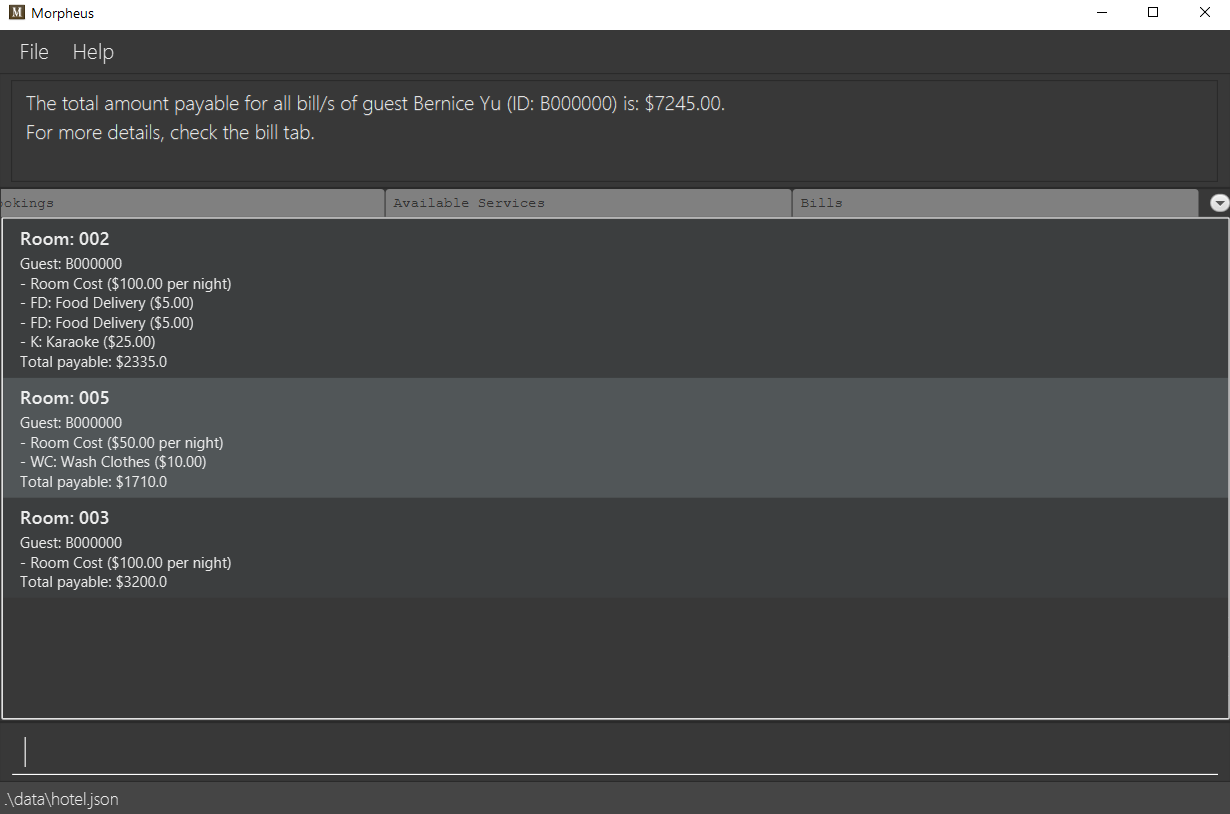
Purpose (Hui Ming)
This Developer Guide describes the architecture and software design of Morpheus. The intended audience is the developers, designers and software testers who wish to understand, maintain & upgrade the desktop application.
Billing System (Hui Ming)
The billing system is designed to aid hotel receptionists in their bookkeeping. It is oversen by the BookKeeper
class, which keeps track of all bills in the hotel and facillitates in the manipulation of bills.
The bills are kept in a UniqueBillList
, which ensures that there are no duplicate bills in the BookKeeper
class.
The structure of the billing system is shown in the class diagram below:

To utilize the billing system, users are provided with the following operations:
-
SetRoomCostCommand
— Sets the cost of a room. -
AddServiceCommand
— Creates a chargeable service. -
ChargeServiceCommand
— Charges a guest for a requested service. -
DeleteChargedServiceCommand
— Removes a charged service from the bill of a guest. -
FetchBillCommand
— Fetches the bill of a guest, including the cost of the room.
The following activity diagram summarizes the typical procedure of billing a guest:

SetRoomCost Command (Hui Ming)
This section goes through the implementation and design considerations of the SetRoomCost
command.
Implementation
The following steps show how the command is implemented:
-
The command from the user is parsed and undergoes checks for the validity of the given
ROOMNUMBER
andCOST
. -
If the parameters are valid,
SetRoomCostCommand#execute(Model model)
is invoked, which checks if the given room exists. -
If the room exists, a
RoomCost
object is created andModel#setRoomCost(Room room, RoomCost roomCost)
is called. -
Room##setCost(RoomCost roomcost)
is then invoked to set the cost of the room by setting the 'roomCost' attribute of theRoom
object. -
If successful, a
CommandResult
object is created to show a success message in the feedback box of the ui.
The sequence diagram below illustrates how the SetRoomCost
command works with the input setrcost rn/001 c/50
:

SetRooomCost
CommandDesign Considerations
Below describes ideas that were considered when designing the command.
-
Alternative 1 (current choice): Store the cost of the room as an attribute in the
Room
object.-
Pros: Application of OOP concepts.
-
Cons: Requires a deeper understanding of the Logic & Model components in order to implement.
-
-
Alternative 2: Store the costs of rooms in a separate list (e.g. as a HashMap) in the Hotel component.
-
Pros: Simple to implement.
-
Cons: Might limit the relationship between the rooms and their costs.
-
AddService Command
The command is also a Hotel Initialization feature and is hence covered above in [Initialization-AddService].
ChargeService Command (Hui Ming)
This section goes through the implementation and design considerations of the ChargeService
command.
Implementation
The following steps show how the command is implemented:
-
The command from the user is parsed and undergoes checks for the validity of the given
PERSONID
,ROOMNUMBER
andSERVICEID
. -
If the parameters are valid,
ChargeServiceCommand#execute(Model model)
is invoked, which checks if the given guest, room and service exist. -
If they exist,
Model#chargeService(RoomId roomId, AvailableService service)
is called which in following callsBookKeeper#chargeServiceToBill(RoomId roomId, AvailableService service)
. -
The bill for the corresponding room is retrieved and
Bill#addService(AvailableService service)
is then invoked byBookKeeper
. -
The service is added to stored list of chargeable objects in the bill and its cost is added to the stored total in the bill.
-
If successful, a
CommandResult
object is created to show a success message in the feedback box of the ui.
The sequence diagram below illustrates how the ChargeService
command works with the input chargeservice i/A000000 rn/001 si/WC
:

ChargeService
CommandDesign Considerations
Below describes ideas that were considered when designing the command.
-
Alternative 1 (current choice): Store the charged services in an ArrayList and the total cost as a double in the bill.
-
Pros: Implementing the removal of charges services would be more direct and simple.
-
Cons: Have to be careful with the calculation of the total cost.
-
-
Alternative 2: Create another class to handle the list of charged services.
-
Pros: Calculation of the total cost would be less prone to errors.
-
Cons: Might cause the design of the application be unnecessarily complicated with many classes.
-