Overview
Morpheus is a desktop application that aims to facilitate the administrative work of hotel services as a hotel room management system. The system will also provide statistics and reports, constructed from the analysis of the details in the user-provided database. Most of the user interaction occurs via a CLI (Command Line Interface).
Summary of contributions
-
Major enhancement #1: implemented check-in/check-out command
-
What it does: This allows the user to check-in or check-out guest into the hotel.
-
Justification: It enables the user to keep track of which room is empty and which room is occupied.
-
Highlights: This enhancement is quite challenging since it is created in the early period which needs more planning.
-
-
Major enhancement #2: implemented extend command
-
What it does: This allows the user to extend the stay of guest.
-
Justification: Guest could probably extend stay if they wanted to. Without creating the bill for the previous stay, extend command allows it to be combined in the same bill.
-
-
Minor enhancement: Added booking ID implementation.
-
Code contributed: [Functional code & Test code]
-
Other contributions:
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Checking in a guest: checkin
(Daniel)
If you want to check in a guest to the hotel from the current date until the end-date, use the command checkin
Format: checkin i/ID rn/ROOM_NUMBER td/TO_DATE
or checkin bi/BOOKING_ID
BOOKING_ID could be shorten by taking only its first 8 characters.
|
Examples:
-
checkin i/G1231231X rn/101 td/2020-12-14
-
checkin bi/a1b2c3d4
Result: Checks in guest with ID A000000
to room 001
until 2020-05-05
. Room 001
will be marked as occupied.
Checking out a guest: checkout
(Daniel)
If you want to check out a guest from the hotel, use the command checkout
Format: checkout rn/ROOM_NUMBER
Examples:
-
checkout rn/003
Result: Checks out the guest from room 003
. Room 003
will be marked as free.
Extend a stay for a guest: extend
(Daniel)
If you want to extend your stay, use the command extend
Format: extend rn/ROOM_NUMBER td/TO_DATE
Example:
-
extend rn/101 td/2020-04-20
Result: Extend the stay of room 101 until 20th April 2020. Room 101
's stay will reflect the updated date.
- Room must be checked in before it can be extended. - The extend period must not clash with other future reservation. |
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Reserve Command
Implementation
This command makes a reservation under the specified guest’s name for the specified duration.
The following steps show how the Reserve command is implemented.
-
The command from the user is tokenized and parsed.
-
If there are no missing tokens,
ReserveCommand#execute(Model model)
is invoked which checks if guest ID, room ID exists in the database and if there are any clashes with other bookings. -
A new Booking object is created and added into the hotel database.
-
If the above is successfully executed, this will return a
CommandResult
object to show a success message.
Design Considerations
Below describes ideas that were considered when designing the command.
-
Alternative 1 (current choice): Reservation is stored in 1 list.
-
Pros: Easy to loop through all reservation to make sure there is no clash between them.
-
Cons: Querying the schedule for a specific room require to iterate through all the reservation.
-
-
Alternative 2: Reservation store for each room.
-
Pros: Each room have their own schedule.
-
Cons: Harder to implement if we want to find an empty room for certain period of time.
-
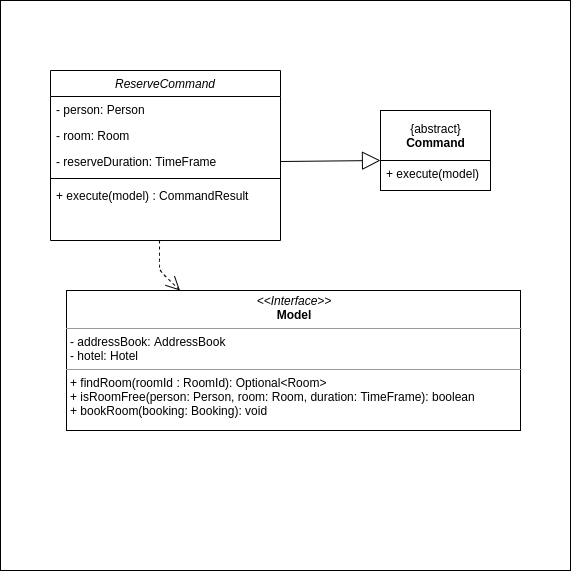
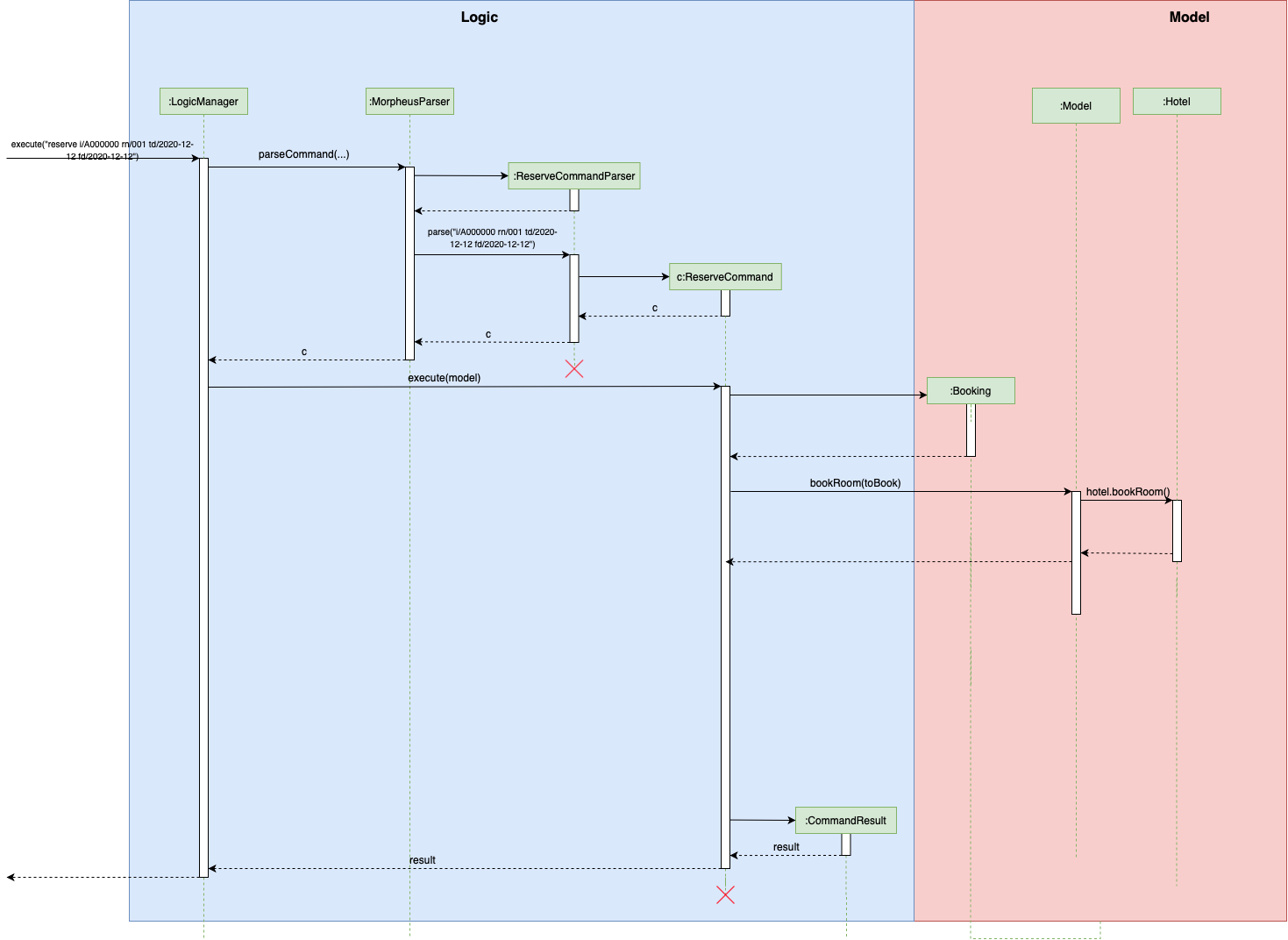
Checkin Command
This command checks in guest either with a room number, guest’s name, and the end date of the stay. Or by providing the booking ID.
Implementation
The following steps show how the CheckIn command is implemented.
-
The command is parsed by
CheckInCommandParser#parse(String args)
into list of pattern there (room number, guest id, end date of the stay or booking id). -
If
BOOKING_ID
exists in the pattern, it will ignore the rest of the pattern and checkin using that booking id instead by creatingCheckInByIdCommand
-
The
CheckInByIdCommand
will invokemodel#findBookingById(String BookigId)
which will create a normalCheckInCommand
-
CheckInCommand
will callmodel#checkIn
will be called. -
The list in the UI will be updated by calling
model#updateFilteredRoomList(Predicate predicate)
-
The room will be charged by calling
model#chargeRoomCost(RoomId roomId, RoomCost roomCost, Stay stay)
. -
If all of the above is successfully executed, this will return a
CommandResult
object to show success message.
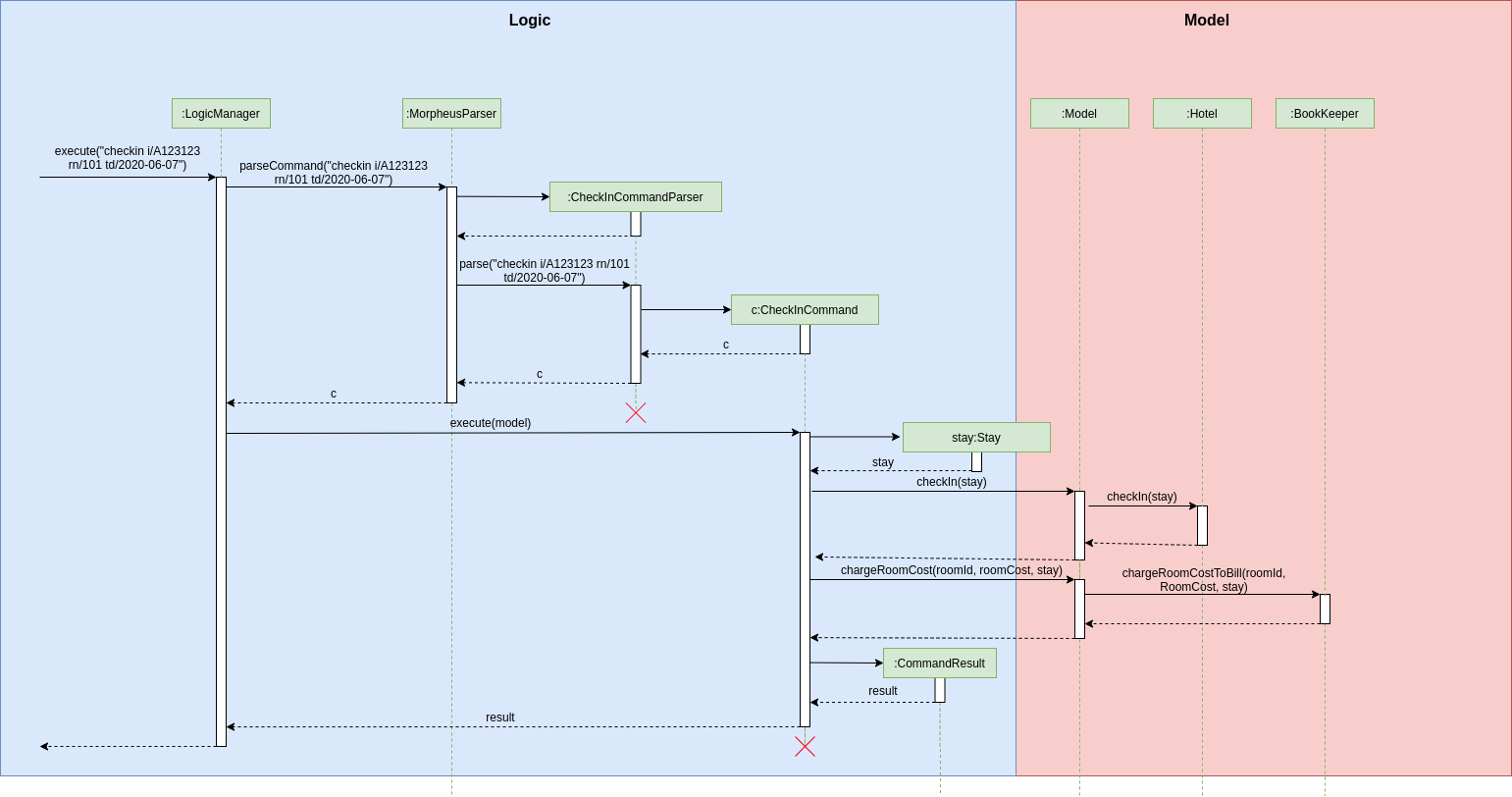
Design Considerations
Below describes ideas that were considered when designing the command.
-
Alternative 1 (current choice): Create a stay object which differentiate between the current stay and reservation.
-
Pros: Could easily get the list of current stay.
-
Cons: Requires more planning since we have to maintain 2 list now (stay and reservation).
-
-
Alternative 2: Store all stay in a reservation object.
-
Pros: Simple to implement.
-
Cons: Could not differentiate between stay and reservation unless there is another instance in the reservation object.
-
Checkout Command
This command checks out a guest from the hotel by providing a room number.
Implementation
The folowing steps show how the CheckOut command is implemented.
-
The command is parsed by
CheckOutCommandParser#parse(String args)
into list of pattern there (room number). -
The
CheckOutCommandParser
will createCheckOutCommand
-
CheckOutCommand
will callmodel#checkOut
will be called. -
The list in the UI will be updated by calling
model#updateFilteredRoomList(Predicate predicate)
-
The room will clean up the previous bill by calling
model#deleteBill(RoomId roomId)
. -
If all of the above is successfully executed, this will return a
CommandResult
object to show success message.
Extend Command
This command extend the booking of a guest by providing room number and end date of the stay.
Implementation
-
The command is parsed by
ExtendCommandParser#parse(String args)
into list of pattern there (room number and end date). -
The
ExtendCommandParser
will createExtendCommand
-
ExtendCommand
will callmodel#extendRoom
to extend the room in the hotel. -
ExtendCommand
will callmodel#chargeExtendRoomCost
to charge the room according to the room cost and number of extra nights. -
If this is successfully executer, this will return a
CommandResult
object to show success message.