Overview
Morpheus is a hotel management system desktop application designed to manage the activities in a hotel. The user interacts with it in a CLI and it has a GUI created with JavaFx. It is written in Java.
Summary of contributions
-
Major enhancement: implementing structure of Hotel
-
What it does: Implementing structure of Hotel, basic objects such as Booking, Charges, Tier, Service.
-
Justification: This enhancement sets foundation for developing Morpheus.
-
-
Major enhancement: implementing hotel initialization
-
What it does: Allows user to initialize hotel with data such as rooms, costs, available services.
-
-
Major enhancement: implementing deleting commands
-
What it does: Allows user to delete guests, rooms, bookings, services from hote.
-
-
Major enhancement: implementing finding commands
-
What it does: Allows user to find guests, bookings, rooms, empty rooms, services from hotel.
-
-
Minor enhancement: Implementing how UI shows response after each command:
-
What it does: Shows response by switching tabs, and filtering details to show that the action has been done.
-
-
Code contributed: [Functional code]
-
Other contributions:
-
Project management:
-
Managed releases
v1.2
-v1.4
(3 releases) on GitHub
-
-
Enhancements to existing features:
-
Wrote additional tests for existing features.
-
-
Documentation:
-
Did cosmetic tweaks to existing contents of the User Guide: #285
-
-
Community:
-
Tools:
-
Integrated a new Github plugin (Travis CI) to the team repo
-
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Overview Of Features
Features can be divided into 5 groups of commands
-
Initialization commands
-
addguest
command adds a guest. -
addroom
command adds a room into hotel. -
addservice
command adds an available service to the hotel. -
setrcost
command sets the cost of a room.
-
-
Service commands
-
reserve
command makes a reservation. -
checkin
andcheckout
command receives and returns customers. -
chargeservice
command charges customers for getting services. -
deletecservice
command removes a charged service from the bill of customers. -
fetchbill
command fetches the bill of a guest.
-
-
Deletion commands
-
delete
command deletes a guest from the hotel database. -
deleteroom
command deletes a room from the hotel database. -
deleteservice
anddeletebooking
commands removes available services and bookings from the hotel database.
-
-
Find commands
-
findroom
command finds room with specific person, or room ids. -
findguest
command finds a guest in the hotel. -
findemptyroom
command finds list of empty rooms in the hotel. -
findbooking
command finds booking ids from names and room ids.
-
-
General purpose commands
-
help
command shows instructions. -
exit
command quits the app. -
clear
command clears all entries. -
switch
command navigate between tabs.
-
Deleting a room by room number : deleteroom
If you want to delete a room from the hotel, use the command deleteroom
.
Format: deleteroom rn/ROOM_NUMBER
Examples:
-
deleteroom rn/101
Result: Deletes room 101
into the database.
Deleting a room by the position it appears in current room tab: deleteroom
If you want to delete a room from the hotel by the index it appears in current room tab.
Format: deleteroom INDEX
Examples:
-
deleteroom 1
Result: Deletes the first room in the list.
Deleting an available service: deleteservice
If you want to delete an available service from the hotel database using the service’s ID, use the command deleteservice
Format: deleteservice si/SERVICE_ID
Examples:
-
deleteservice si/WC
Result: Deletes a service with service ID WC
.
Deletting an available service by the position it appears in current service tab: deleteservice
Format: deleteservice INDEX
Examples:
-
deleteservice 1
Result: Deletes the first room the the list.
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Appendix B: Non Functional Requirements
-
Should work on any mainstream OS as long as it has Java
11
or above installed. -
Should be able to hold up to 1000 persons without a noticeable sluggishness in performance for typical usage.
-
Should be able to hold up to 1000 rooms without a noticeable sluggishness in performance for typical usage.
-
Should be able to handle up to 10000 requests per day.
-
A user with above average typing speed for regular English text (i.e. not code, not system admin commands) should be able to accomplish most of the tasks faster using commands than using the mouse.
-
Should not consume more than 200 megabytes of storage (should optimize encoded data).
-
Should be able to restart without loss of data.
-
Should be able to store back-ups data in case of breaking down.
Appendix A: FindRoomCommand
Implementation
Currently this command only support searching of room full name or/and id number, or room number.
The following steps show how the search guest feature works:
-
The find room command from the user is parsed into a list of pattern contained in the find room command.
-
The find room command then executes and filters the guest list based on the patterns.
The diagram below show how the findroom command store its pattern.
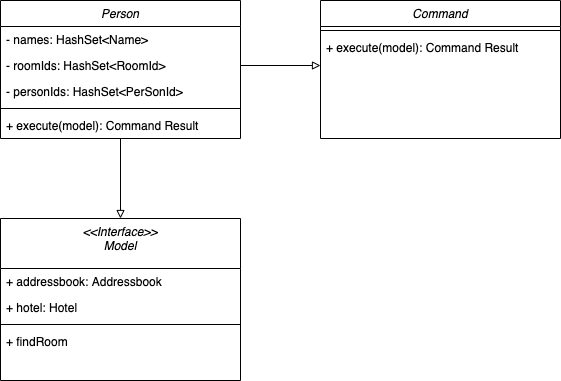
The diagram below shows the execution of the command:
FindBookingCommand
Implementation
Currently this command only support searching of booking full name or/and id number, or room number.
The following steps show how the search guest feature works:
-
The find booking command from the user is parsed into a list of pattern contained in the find booking command.
-
The find booking command then executes and filters the guest list based on the patterns.
The diagram below show how the findbooking command store its pattern.
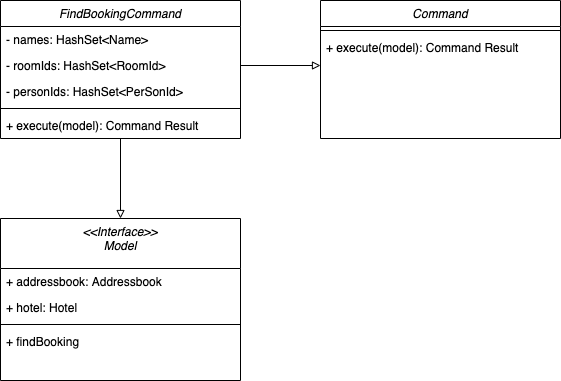
FindEmptyRoomCommand
Implementation
This command support finding empty rooms
The following steps show how the search feature works:
-
The find empty room command from the user is parsed into a list of pattern contained in the find booking command.
-
The find empty room command then executes and filters the guest list based on the patterns.